Print FooBar Alternately
/*
#----------------------------------------------------------------------#
# #
# version 0.0.1 #
# https://leetcode.com/problems/print-foobar-alternately/submissions/ #
# #
# Aleksiej Ostrowski, 2020 #
# #
# https://aleksiej.com #
# #
#----------------------------------------------------------------------#
*/
#include <iostream>
#include <thread>
#include <mutex>
#include <condition_variable>
std::mutex m;
std::condition_variable cv;
int flag = 1;
void task1(const int n)
{
int i = 1;
for(;;) {
std::unique_lock<std::mutex> lk(m);
if (flag == 1) {
std::cout << "foo";
i++;
flag = 2;
lk.unlock();
cv.notify_all();
} else {
cv.wait(lk, []{return flag == 1;});
}
if (i > n) return;
}
}
void task2(const int n)
{
int i = 1;
for(;;) {
std::unique_lock<std::mutex> lk(m);
if (flag == 2) {
std::cout << "boo";
i++;
flag = 1;
lk.unlock();
cv.notify_all();
} else {
cv.wait(lk, []{return flag == 2;});
}
if (i > n) return;
}
}
int main()
{
flag = 1;
std::thread t1(task1, 3);
std::thread t2(task2, 3);
t1.join();
t2.join();
}
Longest Substring Without Repeating Characters
/*
#----------------------------------------------------------------------------------------------#
# #
# version 0.0.1 #
# https://leetcode.com/problems/longest-substring-without-repeating-characters/submissions/ #
# #
# Aleksiej Ostrowski, 2020 #
# #
# https://aleksiej.com #
# #
#----------------------------------------------------------------------------------------------#
*/
#include <string>
#include <iostream>
int max(const int a, const int b) {
if (a > b) return a; else return b;
}
class Solution {
public:
int lengthOfLongestSubstring(std::string s) {
auto len_s = s.length();
if (len_s <= 1) return len_s;
std::string t;
int max_len = -1;
int i = 0;
for (;;) {
auto found = t.find(s[i]);
if (found != -1) t = t.substr(found + 1);
t += s[i];
max_len = max(max_len, t.length());
std::cout << "i = " << i << " s[" << i << "] = " << s[i] << " max_len = " << max_len << " string = " << t << std::endl;
if (++i >= len_s) break;
}
return max_len;
}
};
int main() {
// std::string s = "aabac"; // 3
// std::string s = "pwwkew"; // 3
// std::string s = "bbbbb"; // 1
// std::string s = " "; // 1
std::string s = "aab"; // 2
std::cout << "input " << s << std::endl;
auto S = Solution();
std::cout << S.lengthOfLongestSubstring(s) << std::endl;
return 0;
}
Longest Common Prefix
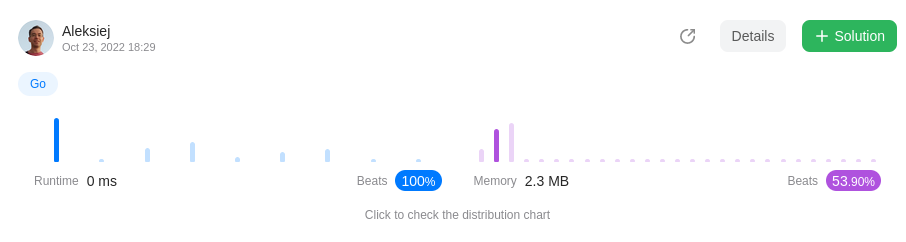
/*
#--------------------------------#
# #
# version 0.0.1 #
# #
# Aleksiej Ostrowski, 2022 #
# #
# https://aleksiej.com #
# #
#--------------------------------#
*/
package main
import (
"fmt"
)
func longestCommonPrefix(strs []string) string {
if (len(strs) == 0) {
return ""
}
maxlen := 201
exclude := 0
for i, el := range strs {
len_el := len(el)
if (len_el == 0) {
return ""
}
if (len_el < maxlen) {
exclude = i
maxlen = len_el
}
}
min_ := maxlen
first := &strs[exclude]
for i, el := range strs {
if (i == exclude) {
continue
}
sum := 0
for j:=0; j < maxlen; j++ {
if ((*first)[j] == el[j]) {
sum += 1
} else {
break
}
}
if (sum == 0) {
return ""
}
if (sum < min_) {
min_ = sum
}
}
return (*first)[:min_]
}
func main() {
var strs = []string{"f"}
fmt.Println(longestCommonPrefix(strs))
}
Roman to Integer
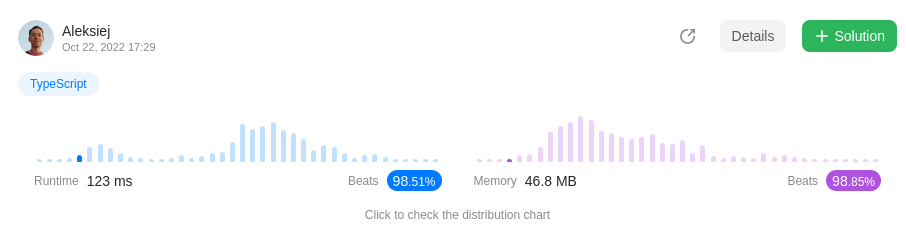
/*
#--------------------------------------------------#
# #
# version 0.0.1 #
# https://leetcode.com/problems/roman-to-integer #
# #
# Aleksiej Ostrowski, 2022 #
# #
# https://aleksiej.com #
# #
#--------------------------------------------------#
*/
function romanToInt(s: string): number {
if (s.length == 0) {
return 0;
}
let res = 0;
let old_level = 1;
let max_level = -1;
//for (const el of s.split("").reverse()) {
for (let i = s.length - 1; i >= 0; i--) {
// console.log(el)
switch (s[i]) {
case "I": {
res = max_level > 1 ? res - 1 : res + 1;
old_level = 1;
break;
}
case "V": {
res = max_level > 2 ? res - 5 : res + 5;
old_level = 2;
break;
}
case "X": {
res = max_level > 3 ? res - 10 : res + 10;
old_level = 3;
break;
}
case "L": {
res = max_level > 4 ? res - 50 : res + 50;
old_level = 4;
break;
}
case "C": {
res = max_level > 5 ? res - 100 : res + 100;
old_level = 5;
break;
}
case "D": {
res = max_level > 6 ? res - 500 : res + 500;
old_level = 6;
break;
}
case "M": {
res += 1000;
old_level = 7;
break;
}
}
max_level = Math.max(max_level, old_level);
}
return res;
}
console.log(romanToInt("MCMXCIV"));
O czym opowiada film Pasożyty?
Wspaniale, że można opisywać problemy klasowe w społeczeństwie poprzez grę aktorów. Nawet dzisiaj, kiedy społeczeństwo jest zupełnie niewrażliwe na twórczość kina. Ponieważ dzięki krótkiemu tik-tok filmiku możesz szybko uzyskać smażony wynik, zupełnie gotowy do połknięcia bez potrzeby filozoficznej refleksji. No co to są za problemy klasowe? Czy podział na klasy nadal jest aktualny? Nie warto tutaj przyglądać się naukowym doktrynom na ten temat. Ponieważ łatwo jest stracić cierpliwość. Bong Joon-ho pokazuje zróżnicowanie klasowe w bardzo prosty sposób. Na przykład reżyser zwraca uwagę na śniadanie dla psów. Dla zwykłych psów — zwykłe jedzenie. Ale dla ukochanego białego psa reżyser podrzuca inne danie — japońskie paluszki rybne. Oczywiście, podczas jedzenia psy muszą patrzeć na siebie nawzajem z poczuciem tego, że jeden z nich panuję nad pozostałymi tylko z tej racji, że jest więcej ukochany. Czy są w filmie pasożyty? Może to bogaci ludzie, którzy nie mogą znieść zapachu ubóstwa? Ponieważ bogaci ludzie nie mogą żyć w społeczeństwie bez pokojówki, osobistego szofera i korepetytorów; pasożytują biednych ludzi, atakują ich jak pchły. Pozostawiam to państwa refleksji.